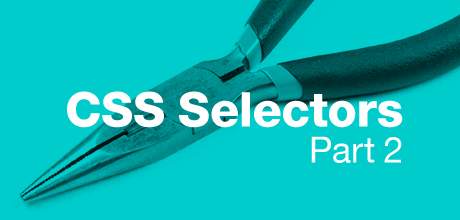
It is the second part of the post dedicated to CSS selectors. In Part 1 I explained the most common and simple selectors like type, ID, class and universal, as well as attribute selectors. In this part I will take a closer look at combinators, pseudo-classes and pseudo-elements.
Combinators
While previously described selectors select an element based on an element type or its attributes, combinator selectors allow you to select an element based on its relationship to other elements on the page. The most important relationships are:
- Ancestor. An element that wraps another element is its ancestor.
- Descendant. An element inside one or more elements is a descendant.
- Parent. A parent element is the closest ancestor of another element.
- Child. An element that is directly enclosed by another element is a child. Second generation and higher element is sometimes referred to as grandchild.
- Sibling. Two elements in the same parent are called siblings, and two elements immediately next to each other are adjacent siblings.
Ok, let’s take a closer look at each of the combinator selectors.
Descendant Selector
Represented by whitespace, descendant selector allows you to create style rules that are effective only when an element is the descendant of another one. The number of elements between the ancestor and descendant doesn’t matter. Consider the following example:
<ul id="main-nav"> <li><a href="">Page Link 1</a></li> <li><a href="">Page Link 2</a> <ul> <li><a href="">Sub-page Link 1</a></li> <li><a href="">Sub-page Link 2</a></li> </ul> </li> <li><a href="">Page Link 3</a></li> </ul>
The following rule matches all five li
elements because each of them has element with an ID equal to main-nav
as its ancestor:
#main-nav li { background: url(icon.gif) no-repeat left top; padding-left: 20px; }
Compatibility: IE 6+, Firefox, Chrome, Safari, Opera.
Child Selector
Child selectors are similar to descendant selectors, but they select only children rather than all descendants. The combinator in a child selector is a greater-than sign (>). For example, if you applied the following CSS rule to the previous HTML fragment, only the list items in the outer list would be given an icon and padding, while list items with the links to sub-pages will remain unaffected:
#main-nav > li { background: url(icon.gif) no-repeat left top; padding-left: 20px; }
Compatibility: IE 7+, Firefox, Chrome, Safari, Opera.
Adjacent Sibling Selector
An adjacent sibling selector uses a plus sign “+” and matches the element that is the immediatelly following sibling of a given element. Consider the following markup:
<article> <h1>Article Title</h1> <p>Some text...</p> <p>Some text...</p> </article>
Say you want to give the first paragraph after each heading different formatting from the paragraphs that follow. The following adjacent sibling selector can help you achieve this:
h1 + p { color: #666; font-style: italic; }
Compatibility: IE 7+, Firefox, Chrome, Safari, Opera.
General Sibling Selector
A general sibling selector is less strict. It matches elements that are siblings of a given element but elements do not have to appear immediately one after another. The combinator in this selector is a tilde sign (~).
h1 ~ p { color: #666; font-style: italic; }
If we applied the above CSS rule to the previous example both p
elements would match the sibling selector h1 ~ p
because both p
elements are siblings to h1
element.
Compatibility: IE 7+, Firefox, Chrome, Safari, Opera.
Pseudo-Classes
A pseudo-class provides a way to select an element based on information that is not specified in the document tree. It always consists of a colon (:) followed by the name of the pseudo-class. No whitespace may appear before or after the colon.
The :link and :visited pseudo-classes
These two pseudo-classes represent the state of links. When unvisited as determined by the browser and its document history, a link can be selected with the :link
pseudo-class, or when visited, it can be selected using the :visited
pseudo-class. For example:
:link { color: #00f; } :visited { color: #999; }
:link
and :visited
are mutually exclusive, that is they cannot be applied simultaneously to the same element. It should also be noted that browsers may treat all links as unvisited, or implement other measures to preserve the user’s privacy.
Compatibility: IE, Firefox, Chrome, Safari, Opera.
The :hover, :active and :focus pseudo-classes
These three can be used to style elements in response to user actions: :hover
is applied when the user’s pointing device is over an element; :active
is applied when an element is being activated by the user; :focus
is applied when an element gains focus. For example, let’s style the states of an input element:
input { border:1px solid #999; color:#999; padding:3px; } input:hover { border-color:#c99; color:#666; } input:focus { border-color:#f00; color:#000; } input:active { border-color:#f00; color:#f00; }
Compatibility: IE 6+ (:focus
in IE 9+), Firefox, Chrome, Safari, Opera.
The :target pseudo-class
URIs with fragment identifiers (represented by the caharacter # followed by an anchor name or element ID) link to a certain element within the document, known as the target element. The :target
pseudo-class selects the target element and lets you style it. Consider the following HTML fragment:
<ul> <li><a href="#section1">Section 1</a></li> <li><a href="#section2">Section 2</a></li> ... </ul> <div id="section1"> <p>Some text...</p> </div> <div id="section2"> <p>Some text...</p> </div> ...
Using the :target
pseudo-class, you can easily highlight the section that the user wants to read:
div:target { border: 1px solid #ccc; background-color: #eee; }
Compatibility: IE 9+, Firefox, Chrome, Safari, Opera.
The :lang pseudo-class
The language pseudo-class allows you to style elements whose content is defined to be in a certain language. For example, article:lang(es)
selects an article element in Spanish.
Compatibility: IE 8+, Firefox, Chrome, Safari, Opera.
The :not(X) pseudo-class
The negation pseudo-class :not(X)
selects all elements except those that are given as the value of an argument (X). For example, the following rule matches all input elements except those with a type
value of "email"
:
input:not([type="email"]) { border: 1px solid #999; }
Compatibility: IE 9+, Firefox, Chrome, Safari, Opera.
The :root pseudo-class
The :root pseudo-class applies one or more styles to the element that is the root element of the document. For HTML documents, this is always the html element.
Compatibility: IE 9+, Firefox, Chrome, Safari, Opera.
The :nth-child and :nth-of-type pseudo classes
The :nth-child(an+b)
pseudo class selects elements that appear at a specific position within the list of its parent’s children. The position is defined via the pattern an+b
, where you replace a
and b
by integer numbers (positive, negative, or zero) of your choice, and n
is a counter starting at 0. The position can also be specified using keywords “even” (same as 2n
) and “odd” (same as 2n+1
).
:nth-of-type
works exactly like the :nth-child
pseudo-class, except that it only considers child elements of the specified type.
Take a look at these two rules:
article :nth-child(3n+1) { color: #999; } article p:nth-of-type(even) { color: #999; }
Since n
starts at 0 and increases by 1 each cycle, the first rule matches the 1st, 4th, 7th, and so on, child elements in an article
element. And the second rule matches only even-numbered paragraphs in an article
element.
Compatibility: IE 9+, Firefox 3.5+, Chrome, Safari.
The :nth-last-child and :nth-last-of-type pseudo classes
These two pseudo-classes accept the same arguments as :nth-child
and :nth-of-type
, except they are counted from the last element, working in reverse. Here is an example:
tr:nth-last-of-type(-n+2) { font-style: bold; }
The count starts at the last tr
element in the table and counts up in reverse order, so the rule is applied to the last two rows in the table.
Compatibility: IE 9+, Firefox 3.5+, Chrome, Safari, Opera.
The :first-child and :last-child pseudo-classes
The :first-child
and :last-child
pseudo-class selectors represent the first and last children elements of some other element. They are equivalent to :nth-child(1)
and :nth-last-child(1)
, respectively.
Compatibility: IE 7+ (:last-child
since IE 9+), Firefox, Chrome, Safari, Opera.
The :first-of-type and :last-of-type pseudo-classes
The :first-of-type
and :last-of-type
pseudo-class selectors represent the first and last children elements of the specified element type. They are equivalent to :nth-of-type(1)
and :nth-last-of-type(1)
, respectively.
Compatibility: IE 9+, Firefox 3.5+, Chrome, Safari, Opera.
The :only-child pseudo-class
This pseudo-class selects an element that has no siblings, i.e. it is the only child of its parent element. Any element selected by :only-child
matches both the :first-child
and :last-child
selectors.
Compatibility: IE 9+, Firefox, Chrome, Safari, Opera.
The :only-of-type pseudo-class
The :only-of-type
pseudo-class matches an element that has no siblings with the same element name. So it matches both the :first-of-type
and :last-of-type
selectors as well.
Compatibility: IE 9+, Firefox 3.5+, Chrome, Safari, Opera.
The :empty pseudo-class
The :empty
pseudo-class targets an element that has no children, including text nodes. Comments and processing instructions don’t count as children. So the selector td:empty
will match only the first td
element in the following markup, as the other two contain a text node and a child element:
<tr> <td></td> <td>Some text...</td> <td><em></em></td> </tr>
Compatibility: IE 9+, Firefox, Chrome, Safari, Opera.
The :enabled, :disabled and :checked pseudo-classes
These pseudo-classes are used to select user interface (UI) elements based on their current state. The :enabled
pseudo-class represents UI elements that are in an enabled state, while :disabled
represents UI elements that are in a disabled state. The following rules will apply different borders to text input elements depending on whether they are enabled or disabled:
input[type="text"]:disabled { border: 1px dotted #999; } input[type="text"]:enabled { border: 1px solid #000; }
The :checked
pseudo-class select UI elements (form controls such as radio buttons, check boxes) that are checked or toggled to the “on” state. For example:
input:checked {outline: 1px solid #f00;}
Compatibility: IE 9+, Firefox, Chrome, Safari, Opera.
Pseudo-Elements
Pseudo-elements target pieces of HTML that do not exist as standalone elements in the document tree. For example, you can use the first-letter
pseudo-element to format the first letter of a paragraph differently, even though that first letter doesn’t have HTML tags wrapped around it.
CSS1 and CSS2 defined pseudo-elements with a single colon (:). To better distinguish pseudo-elements from pseudo-classes, in CSS3 pseudo-elements start with a double colon (::). However, to remain compatible most browsers (except IE7 and IE8) support both versions.
:first-letter pseudo-element
This pseudo-element targets the first letter (or digit) of a block-like elements such as block, inline-block, list-item, table-cell and table-caption. Here is a simple code fragment:
<article> <h1>Article Title</h1> <p>Some text...</p> <p>Some text...</p> </article>
The following selector allows you to style the first letter of each paragraph:
article p:first-letter { font-weight: bold; color: #f00;}
You can also use pseudo-class together with pseudo-element to style only the first paragraph in an article:
article p:first-of-type:first-letter { font-weight: bold; color: #f00;}
Compatibility: IE 6+, Firefox, Chrome, Safari, Opera.
:first-line pseudo-element
The :first-line
pseudo-element selects the first line of a block-like element such as block, inline-block, table-caption or table-cell. The length of the first line depends on a number of factors, including the width of the the element containing the text, the font size, etc.
The following selector will match the first line of every p
element in the previous example:
article p:first-line { text-transform: uppercase; }
Compatibility: IE 6+, Firefox, Chrome, Safari, Opera.
:before and :after pseudo-elements
The :before
and :after
pseudo-elements can be used to insert generated content before or after an element’s content. This content inherits properties from the element in the document tree to which they are attached. For example, say you want to add “Important!” before paragraphs with a class “important
” to make them standout. You can do this using :before
pseudo-element like this:
.important:before { content: "Important! "; color: #f00; }
If you decide to change your special message from “Important!” to, let’s say, “Note.”, then you can change every page on your site with one quick change in your style sheet. However, if the browser doesn’t support this pseudo-element, your special message will be invisible.
It also should be noted that the content generated using :before
and :after
pseudo-elements will not appear to search engines or screen readers, so you shouldn’t rely on it for anything vital.
Compatibility: IE 8+, Firefox, Chrome, Safari, Opera.
::selection pseudo-element
This CSS3 pseudo-element applies to the part of a document that has been highlighted by the user. For example, the following rule makes any selected text white on black background:
::selection { color: #fff; background-color: #000; }
The ::selection
pseudo-element was removed from the current CSS3 draft.
This last selector ends up my overview of CSS selectors. Thanks for reading, and I hope you’ve found some helpful tips!
#1
great job