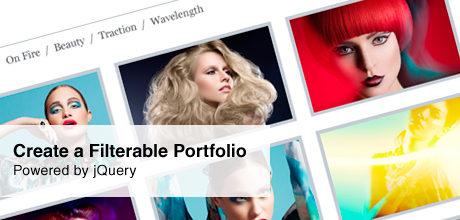
In this tutorial I will show you how to create a filterable portfolio using jQuery. The idea is to display all of your work in a single page that would have a filtering option allowing visitors to filter portfolio items by category. Limiting your portfolio to a single page helps to display all the portfolio items quickly and effectively and the filter makes it easy to navigate.
To filter portfolio items we will use Quicksand plugin. Additionally, we will need Easing Plugin to give advanced easing options and PrettyPhoto plugin to view full-size protfolio items.
The beautiful photos used in the demo are from the portfolio of fashion and beauty photographer Yulia Gorbachenko.
The Markup
Say we have a number of photos in our portfolio and they can be classified in these categories: Beauty, Hannah, On Fire, Traction, Wavelength or Hair. Let’s create a category filter to navigate through our photos. A simple unordered list will perfectly work:
<ul class="filter"> <li class="current all"><a href="#">All</a></li> <li class="beauty"><a href="#">Beauty</a></li> <li class="hannah"><a href="#">Hannah</a></li> <li class="onfire"><a href="#">On Fire</a></li> <li class="traction"><a href="#">Traction</a></li> <li class="wavelength"><a href="#">Wavelength</a></li> <li class="hair"><a href="#">Hair</a></li> </ul>
We assign classes equal to the category name to each list item for later use in jQuery. Additionally, we add one more list item “All” to view all portfolio items. We also assign the class "current"
to this item because we want to show all portfolio items in the default view.
Now when we have our filter let’s move on and create one more list to hold our portfolio items:
<ul class="portfolio"> <li data-id="id-1" data-type="hannah"> <a href="images/hannah_yg.jpg" rel="prettyPhoto[portfolio]"> <img src="images/hannah_yg_thumb.jpg" /> </a> </li> <li data-id="id-2" data-type="hair"> <a href="images/bianca_yg.jpg" rel="prettyPhoto[portfolio]"> <img src="images/bianca_yg_thumb.jpg" /> </a> </li> <li data-id="id-3" data-type="traction"> <a href="images/heather_yg.jpg" rel="prettyPhoto[portfolio]"> <img src="images/heather_yg_thumb.jpg" /> </a> </li> ... </ul>
Each list item contains the thumbnail image with an anchor tag around it. The anchor’s href
attribute defines the URL of the full-size image and the rel
attribute is necessary for the PrettyPhoto plugin that we will use later.
You may have also noticed that every list item has data-id
and data-type
attributes. Both are necessary for the Quicksand plugin. data-id
is a unique identifier and data-type
defines the category to which the portfolio item is assigned. Note that data-*
is a perfectly valid HTML5 attribute.
The CSS
This isn’t a tutorial on CSS, so I won’t go into great detail about how to style the gallery. You can see my stylesheet in the source files attached to this post. I would just like to note that I have used several selectors and properties that are not supported in the earlier versions of IE.
The jQuery
First, we include the latest version of jQuery library and necessary plugins in the head of our document. For the prettyPhoto plugin we also need to include prettyPhoto stylesheet that comes with the plugin:
<link rel="stylesheet" type="text/css" href="css/prettyPhoto.css"/> <script type="text/javascript" src="js/jquery-1.6.2.min.js"></script> <script type="text/javascript" src="js/jquery.quicksand.js"></script> <script type="text/javascript" src="js/jquery.prettyPhoto.js"></script> <script type="text/javascript" src="js/jquery.easing.1.3.js"></script>
Next, when the document is loaded we initialize the prettyPhoto plugin. The prettyPhoto plugin accepts a number of parameters so you can easily customize it:
$(document).ready(function(){ $(".portfolio a[rel^='prettyPhoto']").prettyPhoto({ theme:'light_square', autoplay_slideshow: false, overlay_gallery: false });
Then we add some code to handle the Quicksand plugin:
var $portfolioClone = $(".portfolio").clone(); $(".filter a").click(function(e){ $(".filter li").removeClass("current"); var $filterClass = $(this).parent().attr("class"); if ($filterClass == "all") { var $filteredPortfolio = $portfolioClone.find("li"); } else { var $filteredPortfolio = $portfolioClone.find("li[data-type~="+$filterClass+"]"); }
Quicksand works by comparing two collections of items and replacing them with a nice shuffling animation. By appending .clone()
to our portfolio list and assigning it to $portfolioClone
, we get a copy of the original list items that we will use later.
Once the link in the category filter is clicked, we want to remove the class current
from the list item that has it and to determine which link has been clicked by taking the class value and assigning it to the $filterClass
. In the case if the “All” link has been clicked, we want to show all of the portfolio items. Otherwise, we want to show only those list items that have data-type
attribute with a value containing $filterClass
.
$(".portfolio").quicksand( $filteredPortfolio, { duration: 800, easing: 'easeInOutQuad' }, function(){ $(".portfolio a[rel^='prettyPhoto']").prettyPhoto({ theme:'light_square', autoplay_slideshow: false, overlay_gallery: false }); }); $(this).parent().addClass("current"); e.preventDefault(); }) });
Finally, we call quicksand
. $filteredPortfolio
which was assigned in the if statement contains filtered portfolio items that are to be displayed instead of the original portfolio items. We can also specify how long the animation will take, the easing type for the animation (don’t forget to include jQuery Easing Plugin for this) and other optional parameters. As the last argument we include a callback function to apply prettyPhoto on newly cloned portfolio items as well.
In the last lines of code we add the class current
to the parent of the clicked link and call preventDefault()
to prevent the browser jump to the link anchor.
With that our filterable portfolio is finished. I hope you enjoyed the tutorial and find it useful! I tried to explain everything as best as I can and if you have any questions, please feel free to ask.
Follow us on Twitter, or subscribe to RSS Feed for more tutorials and posts.
#1
do you have live examples of what this page would look like? Im trying to see if it might work for my writing portfolio.
#2
There’s a demo button at the beginning of the post or you can follow this link .
#3
Nice script Asta, is it possible to also have a the navigation menu with a radio button tick box ie. you could tick hair and beauty and see both, but not the others. I know jquery can filter like this but not sure how to combine. This would be a nice feature. I am thinking about using this for my portfolio.
Thanks,
Martino
#4
Thanks Martino!
If you want one portfolio item to be classified in several categories, you can add categories separated by whitespace characters like this:
But I didn’t think of the possibility to select several categories in the filter at once, because in my opinion it’s not a very useful feature in this case. If you had several criteria to filter your portfolio, for example, category (logo, print,..) and year (2009, 2010, 2011), then you could use multiple filters. Here’s an example with multiple filters and Quicksand. I hope it will help you.
#5
I’m curious to know how this can be incorporated into a WordPress template.
#6
Kevin, you could set up custom post type “portfolio” and custom taxonomy “categories” and then add custom template to display portfolio gallery. I’m currently developing a theme that will have filterable portfolio and will write a post about this in a couple of weeks.
You can read more about post types in WP Codex.
#7
That’s a great jquery gallery Asta. I just wanted to know if it was possible to add a image description to the large image when it pops up. I know html and css but im very new to jquery.
Thanks
James
#8
Thanks, James :) To add a description you just need to add the title attribute to an a tag:
You can also display the title of the image when it pops up. Just change the value of the parameter show_title to true in the script.js file (lines 15, 52). Check jQuery prettyPhoto documentation for more options.
#9
Hi Asta,
Thank you very much for your quick reply. I will put a backlink to your website once I have the gallery up and running.
Cheers
James
#10
Thanks for the time you put into this.
I spent all day looking for a slick portfolio filtering plugin, and this is the first one that did what it claimed to do without three hours of headaches.
Great work!
#11
Glad to hear that! Thanks :)
#12
I’ve noticed something in the demo with regard to prettyPhoto’s available themes (http://www.no-margin-for-errors.com/projects/prettyphoto-jquery-lightbox-clone/documentation/). Examples of built-in prettyPhoto themes are pp_default, light_square, dark_rounded, light_rounded, etc:
$(document).ready(function(){
$(“a[rel^='prettyPhoto']“).prettyPhoto({
theme: ‘light_rounded’
});
});
If I apply one of these prettyPhoto themes to your Portfolio filter demo, the theme will only be applied BEFORE a filter is used. However, when a filter is clicked and and the thumbnails are reconfigured, the applied theme is no longer recognized or applied.
My Javascript skills are non-existent, so I’m unable to even guess what might be going on here.
Any ideas why the theme gets reset when the filter is used?
If posting a demo is useful, let me know and I am happy to post it so you can see what I mean.
#13
Okay, I think I’ve got it sorted now. Seems that in the script.js file, the ‘light_square’ theme gets applied by default (line 12) and again when calling quicksand (line 49). By changing both of these values to the theme I want, things worked great.
Thanks!
#14
Yes, you have to apply prettyPhoto on newly cloned items as well. Glad you got it sorted out :)
#15
I love it!
Having a little issue though – I would like to use rollover captions for the images, and I can’t seem to get this to play with other jQuery plugins like Captify…
I’m no coder, just more of a dabbler!
Any suggestions or nod in the right direction?
#16
Hi, sorry for the delay, I have been very busy lately. Hope this would still be useful for you. To add just a simple caption rollover you could do like this.
Add caption to each list item:
Then add this to CSS file:
And then edit script.js file. Find this piece of code(lines 3 and 42):
and replace with:
If you want to use a jquery plugin to add a fancier effect, just read carefully the documentation of the plugin.
#17
Hi, first I want to said thanks for this post, really great.
I have a question about the caption, it´s possible to hide it on mouse out or something?
Thanks for your help.
#18
Hi, you’re welcome :) My comment above explains how to add a simple caption rollover: captions shows up on mouse over and disappears on mouse out.
#19
The whole filterable function is amazing–sleek and efficient. I do have one question regarding the additional rollover captions: It only seems to work on the “ALL” set of photos. If one chose a filter, then the rollover caption does not appear.
Moreover, once the user choose “ALL” again, the rollover do not appear also, unless one reloads the webpage. I just wonder if anything can be done about it? I checked into the script.js and it seems the second set of “on hover” commands needs to be modified (which I tried) but still wouldn’t work.
peace,
Richard
#20
You need to add rollover captions on newly cloned portfolio items as well. See my comment above.
#21
Hi, do we have permission to use this tutorial for commercial work?
#22
Yes you do :) I am glad this tutorial was useful for you.
#23
thanks so much for the tutorial. is there a way to have multiple pages for each large pop up? for example, if i wanted to show 3 or 4 images of the same project.
#24
You are welcome :)
First, add more images to each list item (project):
Pay attention that rel attribute must be unique for each list item (project).
Then to show only first image of each project and hide all others, add this line to the end of CSS file:
That's it!
#25
The “display: none” doesn’t seem to work for some reason
#26
Hi,
I want to remove “All” tag and by default i want to filter it on page load on my first catagory?
Is it possible.
#27
This worked for me ..
Remove this line
All
and add$(function () {
at the bottom of document ready section in the javascript file$("li.beauty a").click();
});
#28
Hi – Thanks for creating this simple yet extremely useful plugin! I noticed the images are taking a bit of time to load. Any idea if this is due to slowness in prettyPhoto or else the host web server?
Thanks,
-Sid
#29
You are welcome :) prettyPhoto needs a bit of time to load.
#30
Hi Asta first of all thanks for this great tutorial and your time. this tutorial help me alot and now i want to add sub level of categories and not know how to do this plz can you help me how to do this
I uploaded a demo image what i want..
http://www.megagamezone.com/wp-content/uploads/categorymenu.png
upper menu is main category menu and second menu is all categories under main menu when someone click on specific sub category then all portfolios in that category will be shown..
Thanks in advace..
#31
Hi, sorry, but I am very busy at the moment. Maybe later I will make a tutorial on how to do this. For now here is an example with multiple filters http://www.danieltulp.nl/work/quicksand-multiple.htm. Hope this will help you :)
#32
hi asta
thank you for the great tutorial i want to change the image background color which is white at the moment can you please or someone tell me how can i change it please
email me on [email protected]
thank you
#33
Hi, glad that it was helpful for you. Take a look at the CSS file, line 53. A white 3px border is added around the links. Change the color or edit other CSS rules according to your needs.
#34
Hi Asta,
I want to remove “All” and by default i want to load portfolio with my first catagory like “Beauty” ?
Is that possible to filter on page load.
Please let me know As soon As possible
#35
Hi, it’s possible. Call
quicksand
on page load and setduration
parameter to 0.#36
can you please describe me with example i have try but its not working.
#37
Hi
Please give me script change with example…………
So i can do it becos i m very much new to Jquery.
#38
pagination?
#39
To my knowledge it works only without pagination. But there is a plugin Quicksand-And-Paginate-Plugins-Joint. Haven’t tested it myself.
#40
Hi! Im trying as well to land directly to one of the categories when the portfolio loads (i’ve plenty of images and dont want to load all of them by default).. you reply something above “call quicksand on page load ans set duration parameter to 0″.. but dont know exactly where and how to code it.. hope you can help me out..
Thank you!
#41
Aciu labai uz si koda. Kaip tik tai ko ieskojau. Panaudosiu savo portfolio!
#42
sorry for the typo: panauduosiu. :)
#43
Hi Asta,
I’ve been playing around with this fantastic system and it does everything I want! The only issue I am having is that I’m using title=”…” to hold a description of the image so that it appears below it in the lightbox. Is there anyway I can do this but prevent the text from appearing as a tooltip when it’s hovered over before the click that brings up the lightbox?
Thanks again!
#44
Dear Asta,
I just wanted to say thank you for this tutorial. I’m not a web designer but do try to make my own. It’s quite hard to put certain elements together and scripts clash, I’ve spent the past week trying to make Quicksand and a gallery script work, but without perfect success… then I stumble across this tutorial – complete and perfect in every sense and it puts a smile on my face that someone will go the length of putting it together and make it available for others.
So THANK YOU for your effort :)
#45
Thank you for the great tutorial! By far the easiest one to follow on this topic! I have implemented the code on http://www.thinkcreatelive.com/graphicdesign. Love how it works and looks! I am, however, having an issue working Fancybox into the code, which is what I’m currently using on my home page. My file for the portfolio images is very lengthy but here’s how the first part is structured:
$(document).ready(function() {
$("a.fancybox").fancybox({
'titlePosition' : 'inside'
});
$("#graphicgallery1").click(function() {
$.fancybox([
{
'href' : 'images/graphic/mag1.jpg',
'title' : '1/4: Cover design for a twice-yearly style magazine for WITNESS, a nonprofit human rights advocacy group.'
},
{
'href' : 'images/graphic/mag2jpg',
'title' : '2/4: Image heavy spread focusing on the work of filmmaker Shirin Neshat.'
},
{
'href' : 'images/graphic/mag3.jpg',
'title' : '3/4: Multicontent spread showing the conclusions of two articles and a reader’s buying guide.'
},
{
'href' : 'images/graphic/mag4.jpg',
'title' : '4/4: Text heavy spread focusing on Google’s involvement with the Crisis in Darfur.'
},
], {
'padding' : 10,
'transitionIn' : 'none',
'transitionOut' : 'none',
'type' : 'image',
'titlePosition' : 'inside',
'changeFade' : 0
});
});
This works perfectly on my current site and I hope it can work with this sleek new layout :) Any help you could provide is greatly appreciated! Love your work/blog and I’m already following you and Tomas on Twitter! Thank you for your time!
#46
Hi Asta,
Thank you for this tutorial, it works great,
I’m an architect and planning to use it on my website, if you allow me to
I have only one question,
I want to remove “the prettyphoto plugin” and instead launch an event that by clicking on a picture it would take me to another page where all the information of the targeted picture is
Thanks
#47
Hi, you’re welcome :) Feel free to use it on your website.
To remove prettyphoto, first remove the link to the jquery.prettyPhoto.js file in the head of index.html (line 10):
Then in index.html change the
href
attribute value of the anchor tags and removerel
attribute:In script.js file find and delete this bit of code (lines 11 and 48):
Good luck!
#48
Hello Asta,
Great tutorial you have here!
Although, I’m having a problem with my atempt to replicate it.
The UL with the category filter disappears after selecting one of the items… Could you guide me to the best solution?
Thanks in advance and keep up the great work ;)
#49
Oh I forgot to mention the link… It is
Here
#50
i was wondering why if the thumbs takes 3 columns the align gets messy. Your demo have the same problem.
#51
There is a rule in my stylesheet that removes the margin from every 4th list element:
Edit the stylesheet according to your needs.
#52
thanks! you save my day
#53
Great work! :) I’ve been looking everywhere for a tutorial explaining how to merge prettyPhoto and Quicksand.
I had one question I was hoping you could help me with – after I filter the portfolio and open an image in prettyPhoto the title contained in the alt tag no longer displays at the top of the prettyPhoto window.
I’m a rookie when it comes to Javascript, any idea how to fix this?
#54
Hi Asta,
Can you add a code of page scroll easing? Because if there are lots of portfolio to showcase, it’s doesn’t look right if it just re-size right-away when switching a category showing only 3 projects.
Thanks,
#55
And by the way, how to fix if this line “#!prettyPhoto” appearing at the last line in the address bar after using the prettyPhoto?
#56
Hi Asta,
I’ve fixed the line “#!prettyPhoto” that show’s up in the address bar. And now what’s left is the easing for the page. :(
Thanks,
#57
Hello Asta, thanks for the great tutorial.
im a newbie and having some trouble implementing this into my site, and i was hoping you, or someone else could help out.
for some reason the larger images are not showing once a thumbnail has been selected, but i cant work out why this might be happening? any advice would be great.
regards
pk
#58
also, i forgot to ask. how would i amend the images to display inline info instead of images?
#59
Great Stuff!
I am very new to all of this – I know next to nothing. I have been combing through your code looking for a way to list the objects only vertically, creating a single column. I can achieve this by shrinking the stage area (or adjusting the window). Is there a way to do this?
Thanks!
#60
Hi Asta
Thank you for your great tutorial, it does just what I was looking for my photo portfolio. The only problem I found is that I’m trying to combine horizontal and vertical thumbnails without messing everything. Is there any way the thumbnails could be contained on an square invisible box In which I could center my vert & horz images. Could you help me on this?
Thank you in advance
#61
Hi Asta
Thanks for the fantastic tutorial, I’ve just about got everything working at this point. Is it possible to remove the ‘all’ category from the portfolio? I saw your reply to another comment that talked about calling quicksand, but I’m not quite sure how to implement that. Any help would be greatly appreciated.
Cheers!
#62
Hi,
@ Ali, I think that you just have to delete that line in the filter unordered list :
All
And keep the others.
Then, I would like to thanks you Asta for this tutorial that helps me to resolve a problem I had building my portfolio, so again Thanks a million !!!
I would like to know if there’s a way that quicksand arrange each element of the portfolio randomly ? I’m not sure if they’re arranged by order of appearance in the Html code, or alphabetically as I just started to fullfill my portfolio, but is there a way to do display elements randomly ?
Thanks in advance.
Regards.
Panga.
#63
@Ali
Sorry for the previous message, the line to delete is :
All
Regards.
#64
“All</li"
#65
Sorry,
I don’t want to spam this page, but I do not success to display the line correctly, It makes a link each time, so Ali, delete the second line in the first code in the tutorial and it’ll be fine.
#66
Hi! Could you tell me how to add this filter effect to my wordpress portfolio page (duotive 2 theme)?
Thank you so much!
#67
hello. great work. i know this has been answered, but being new to jquery, i was unable to follow.
please let me know how to remove “all” and immediately have the code load my first category in the portfolio.
My “all” list is simply too long and i dont want it to show up at all.
thank you!!!
#68
this portfolio is increble. it might just solve all of my problems!
the tutorial is really clear as well.
just wondering, is there any way I could add videos as well to the gallery? I realize that this might be a little hard, but I just wanted to ask before giving up straight away on this;)
#69
just resolved this! sorry for the silly question.
#70
Great tutorial. Exactly what I was looking for. Thank you so much for pulling it all together and for being so responsive in the comments section. Is there a way to link to the already filtered results of a particular category, rather than having to show “All” by default? I love the “All” option, but I also would like to be able to link people directly to an already filtered page. Thanks!
#71
Is there a simple way to change the filter trigger to a pop-up list?
#72
hey,
first of all, congrats for the amazing work. I love this javascript so much! In fact, I used it on my website as well:
http://frenkiprod.altervista.org
there’s only one problem though: it seems like it works perfectly on mozilla but not on safari. Did I do something wrong?
#73
Thanks, as far as I can see it works on safari as well.
#74
Really good tut, been looking for this for ages.
Got it all working, bar 1 or 2 small problems:
in the demo the thumbs are in rows of 4, i have larger images that show 3 per row, however after the 4th image there is no margin between the 4th and 5th, 8th and 9th etc.
It doesnt seem to be css related and still works, it just messes about with the spacing.
Also i have an portfolio class already, i have temp changed it to get this working, only i would like to change it back for the sake of file name consistancy, ergo i need to change the .js and your css references to “portfolio” to say “pitem”, only this seems to kill the js. I am not really sure what to rename in the script.js
#75
Thanks! Regarding your first issue, there is a rule in css stylesheet:
This pseudo-class selector matches every fourth element, so change it according to your needs.
Regarding the second issue, you have to change references on lines 4, 11, 19, 36, 42, 48 in script.js file and lines 51, 52, 53, 54 in style.css file.
#76
Thanks so much, worked like a charm, i had not come across “nth-child() before but i should have realised when reading it!
Regarding the second problem, it was as i thought, i foolishly ‘forgot’ to change the
Great site, thanks for the quick responce
#77
You’re welcome :) Good luck!
#78
This is a wonderful tutorial and outcome, I have one question regarding linking though. How would I go about removing prettyphoto and instead having the images link using their
Example
value? Thank you once again!#79
Thanks :) First, you need to edit the list of portfolio items in html file (starting from line 41). Change the href attribute value to your specific url and remove rel attribute. For example:
should be:
Next, edit script.js file. Remove this block of code (lines 11 and 48):
Lastly, remove link and script tags in html file (lines 7 and 9) that include prettyPhoto stylesheet and javascript file:
You can delete prettyPhoto.css and jquery.prettyPhoto.js files as well.
And that’s it. Good luck with your project :)
#80
Thanks for this!
#81
Thank you so much for your wonderful tutorial and plugin! I am having some problems implementing this on a WordPress based site. Everything works fine, but the first image keeps going to the right. Here is a screenshot of what I mean.
Click me!
#82
You’re welcome :)
Since you apply
float: left
CSS rule to your category filter and portfolio items, you need to clear floated elements.#83
Where exactly would I put the clear property? I tried putting it in the css in the portfolio items section, but it made everything block items. I then tried setting the display to inline, but that just messed things up.
#84
Nevermind, I figured it out :). Thanks for your help!
#85
Hi, just wondering if its possible to replace PrettyPhoto with Shadowbox? I tried and the filters wouldn’t work…
#86
Hi, I’ve placet the code within 2 other divs and it wouldn’t quite work ie ‘all’ wouldn’t display all images. Any suggestion why?
#87
First off – Great tutorial! This code is very slick.
I’m wondering if there is a way to use hash tags so that I can have links elsewhere on my site that directly go to a particular filter. Or if not how could I apply current so that the default is something besides All (in this case I’d be making multiple pages with the gallery and just changing where current is applied – not ideal)?
Thanks so much.
#88
Nice tutorial, Thank you so much.
But i think i made some mistake some where. I have integrated it into joomla. and i think it is not working properly. Checkout the animation when switching categories.
here
#89
Wow thank you so much for sharing this!
I tried to open an iframe instead of an image but did not succeed. So now I try to add a link to the description.
Adding a link in html charachters works but … i want to open the link (in the image description) in a new window …
Must I keep on dreaming or is there a way?
Either opening an iframe or adding a link with target in the description …
#90
Hi, works with .swf or .flv too?
thanks
M
#91
Awesome tutorial, Thank you so much!
But i have some problems with viewing the enlarged image on IPAD. After turning around the ipad to vertical and horizontal way to view the pop out image, it won’t show up in the center.
Any suggestions?
Thanks again!