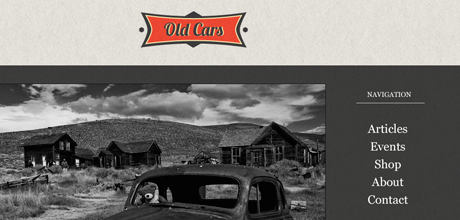
In this short tutorial we are going to build a nice and eye-catching animated navigation menu with the help of jQuery. We will need hoverIntent and BackgroundPosition jQuery plugins, as well as sprite image for our menu items.
Let’s start with the markup.
The Markup
To keep semantic code our menu is organized as a simple unordered list:
<ul> <li id="articles"><a href="#"><span>Articles</span></a></li> <li id="events"><a href="#"><span>Events</span></a></li> <li id="shop"><a href="#"><span>Shop</span></a></li> <li id="about"><a href="#"><span>About</span></a></li> <li id="contact"><a href="#"><span>Contact</span></a></li> </ul>
Our list items will only be displaying images (with CSS sprite method) but we do not want to leave them blank because of search engines. So we add a span inside the anchor tag of each list item. We will hide those spans form the user’s view in our stylesheet. We also assign an id to each list item for later use in CSS and jQuery.
The CSS
We will use CSS sprite method for our menu items.
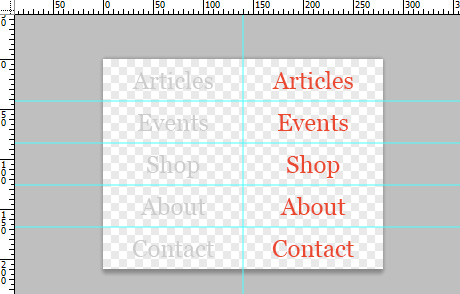
We load a single background image for all of our menu items and then give individual ones their own coordinates. The id that we assigned to each list item earlier allows us to target each item individually.
ul { list-style: none; margin: 0 auto; } ul li a { display: block; height: 42px; background: url(../images/nav.png) 0 0 no-repeat; } #articles a { background-position: 0 0; } #events a { background-position: 0 -42px; } #shop a { background-position:0 -84px; } #about a { background-position:0 -126px; } #contact a { background-position:0 -168px; }
Finally, let’s add some jQuery magic.
The jQuery
First, we include the latest version of jQuery library and necessary plugins in our html document:
<script type="text/javascript" src="js/jquery-1.7.1.min.js"></script> <script type="text/javascript" src="js/jquery.hoverIntent.minified.js"></script> <script type="text/javascript" src="js/jquery.backgroundPosition.js"></script>
hoverIntent jQuery plugin attempts to determine the user’s intent. It was derived and works like jQuery’s built-in hover. However, instead of immediately calling the onMouseOver function, it waits until the user’s mouse slows down enough before making the call. Thus it delays or prevents the accidental firing of animations.
The other jQuery plugin, BackgroundPosition, adds the ability to do background-position animations since animating multi-value properties like background-position is not supported in jQuery by default.
Next when the document is loaded we call hoverIntent method on our menu items. As with jQuery built-in hover, we need to define two functions. First will execute when the mouse pointer enters the element and the second when the mouse pointer leaves the element.
When the mouse pointer enters menu item we animate the horizontal position of its background image to the left. We repeat animation for several times to create a nice bouncing effect. When the mouse pointer leaves menu item we return its background image to the original position and again we repeat animation for several times to achieve a bouncing effect.
$(document).ready(function(){ $('li').hoverIntent( function(){ navitem = $(this); if (navitem.attr('id')==='articles'){ypos1=0;} else if (navitem.attr('id')==='events'){ypos1=-42;} else if (navitem.attr('id')==='shop'){ypos1=-84;} else if (navitem.attr('id')==='about'){ypos1=-126;} else {ypos1=-168;} navitem.children('a').stop().animate( {backgroundPosition:'-150px '+ypos1+'px'}, 220, function(){ navitem.children('a').animate( {backgroundPosition:'-137px '+ypos1+'px'}, 64, function(){ navitem.children('a').animate( {backgroundPosition:'-140px '+ypos1+'px'}, 32); }); }); }, function(){ navitem2 = $(this); if (navitem2.attr('id')==='articles'){ypos2=0;} else if (navitem2.attr('id')==='events'){ypos2=-42;} else if (navitem2.attr('id')==='shop'){ypos2=-84;} else if (navitem2.attr('id')==='about'){ypos2=-126;} else {ypos2=-168;} navitem2.children('a').stop().animate( {backgroundPosition:'6px '+ypos2+'px'}, 220, function(){ navitem2.children('a').animate( {backgroundPosition:'-3px '+ypos2+'px'}, 64, function(){ navitem2.children('a').animate( {backgroundPosition:'0 '+ypos2+'px'}, 32); }); }); } ); });
By this point our animated navigation menu is finished. Feel free to modify it according to your needs and use it to spice up your own websites. :)
Follow us on Twitter, or subscribe to MazeofMinds RSS Feed for more tutorials and posts.
#1
Thanks for the script and your time :)